[검색엔진] django로 Basic 검색 웹사이트 만들기 (Basic Filtering)
2020. 6. 25. 16:35ㆍ노트/Django : 웹
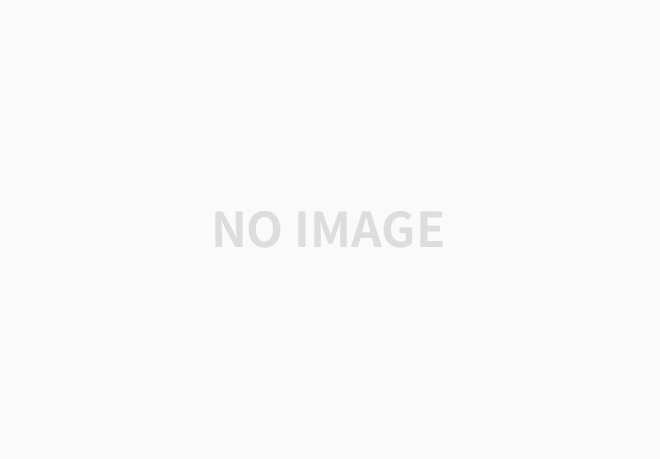
1. 가상환경에 장고 환경 세팅 (Terminal에 입력)
$ python -m venv venv
$ source venv/Scripts/activate
$ pip install django==3.0.3
$ pip install shell
$ pip install cities
2. 프로젝트 생성 (Terminal에 입력)
$ django-admin startproject citysearch_project .
$ python manage.py migrate
$ python manage.py startapp cities
3. Apps 설치
- settings.py > INSTALLED_APPS > 'cities.apps.CitiesConfig' 추가
# citysearch_project/settings.py
INSTALLED_APPS = [
...
'cities.apps.CitiesConfig', # new
]
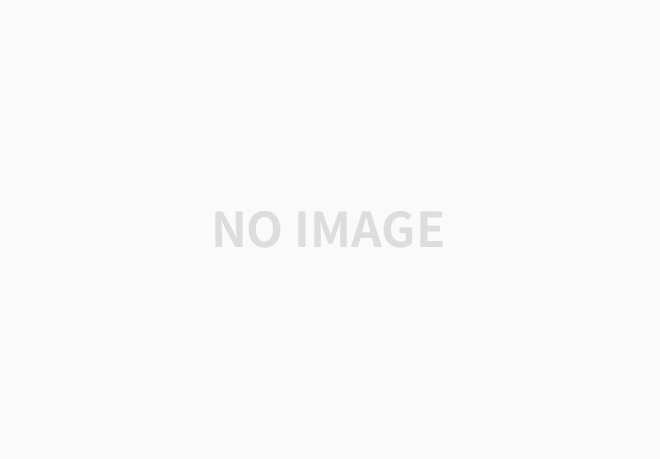
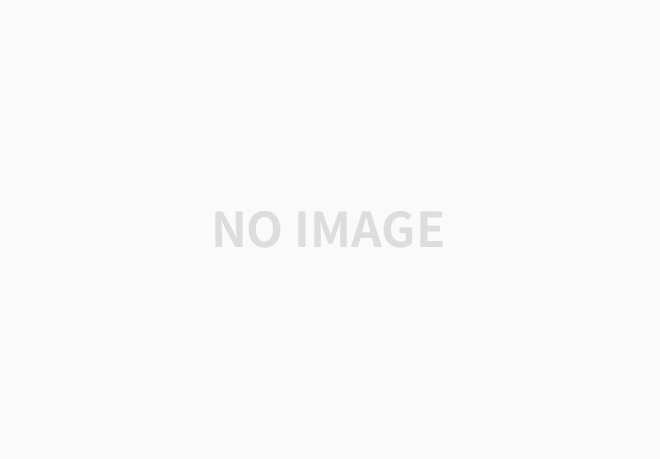
4. Model 생성
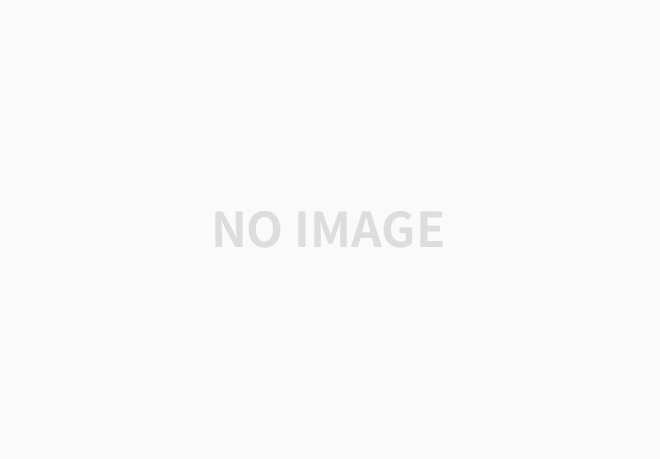
# cities/models.py
from django.db import models
# Create your models here.
class City(models.Model):
name = models.CharField(max_length=255)
state = models.CharField(max_length=255)
class Meta:
verbos_name_plural = "cities"
def __str__(self):
return self.name
- model을 생성했으므로 migration 하기
$ python manage.py makemigrations cities
$ python manage.py migrate
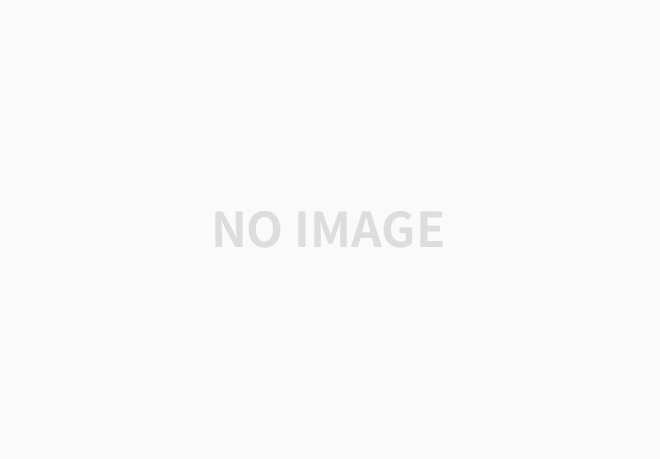
5. 관리자 계정 만들기
$ python manage.py createsuperuser
Username : student
Email address :
Password : 1234
Password(again) : 1234
...~ create user anyway? [Y/N]: Y
Superuser created successfully
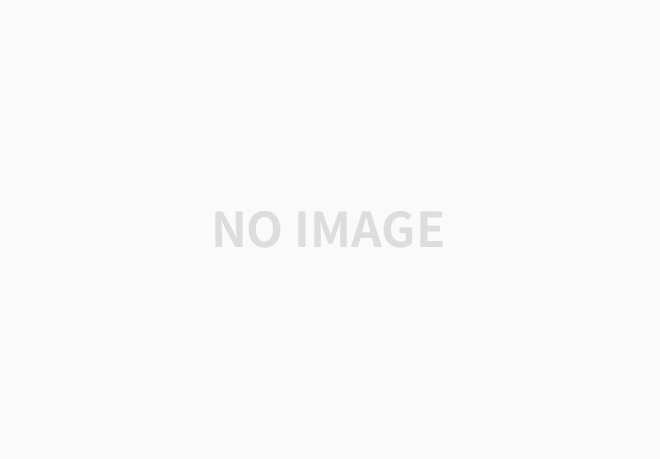
- city > admin.py 파일 업데이트 ( 관리자 페이지 뜨도록 하기 위해서)
# cities/admin.py
from django.contrib import admin
from .models import City
# Register your models here.
class CityAdmin(admin.ModelAdmin):
list_display = ("name", "state",)
admin.site.register(City, CityAdmin)
# 장고에서 웹서버 실행
python manage.py runserver
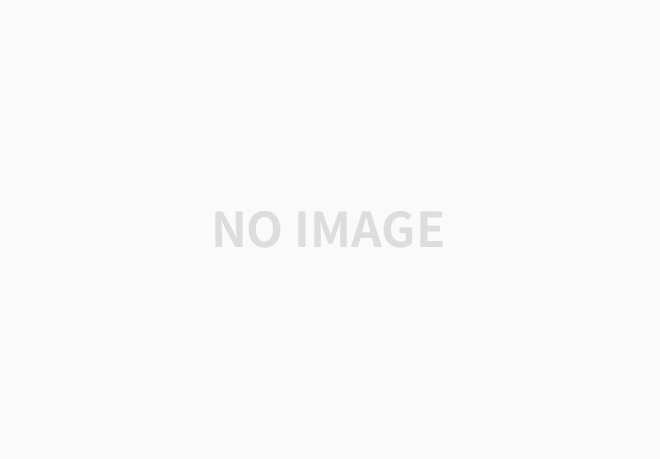
- 관리자 페이지 확인 가능
6. 홈페이지와 검색 결과 페이지 만들기
- 검색결과를 불러올 urls 경로를 추가하기 "citysearch_project/urls.py"
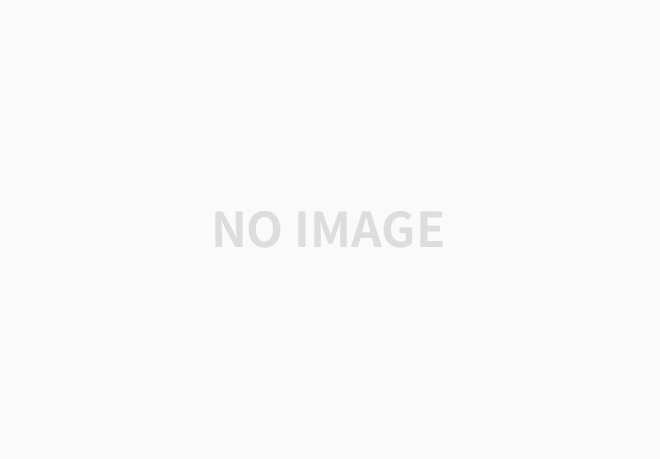
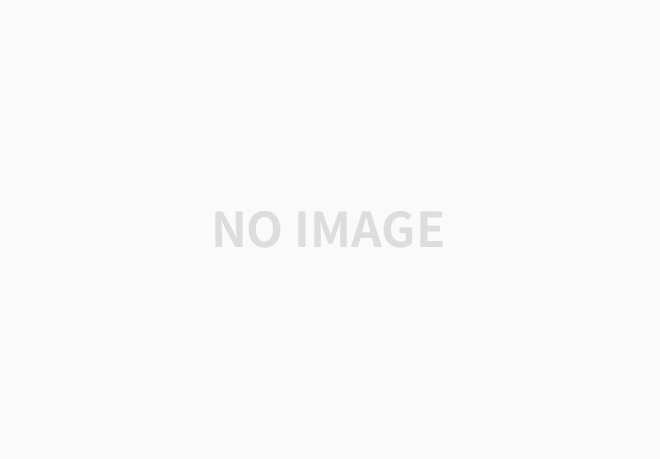
# citysearch_project/urls.py
from django.contrib import admin
from django.urls import path, include # new
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('cities.urls')), # new
]
- cities 폴더에도 urls.py 경로를 표시하기 위해 터미널에 아래의 명령어 터미널에 입력하기
$ touch cities/urls.py
- 파일 생성 확인
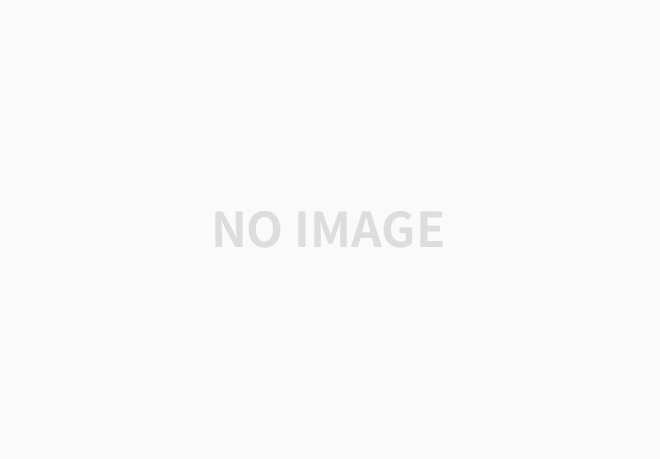
- 검색결과를 나타낼 url 경로 입력하기 "city/urls.py"
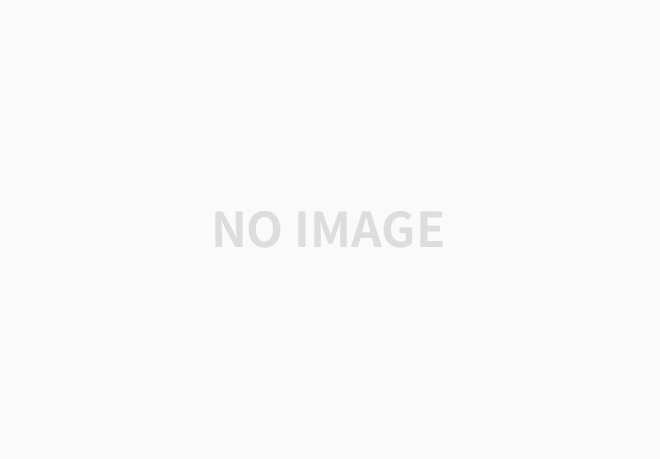
# cities/urls.py
from django.urls import path
from .views import HomePageView, SearchResultsView
urlpatterns = [
path('search/', SearchResultsView.as_view(), name='search_results'),
path('', HomePageView.as_view(), name='home'),
]
7. 홈페이지 view 와 검색결과 view 창 만들기
- cities > views.py 에 아래의 코드 입력
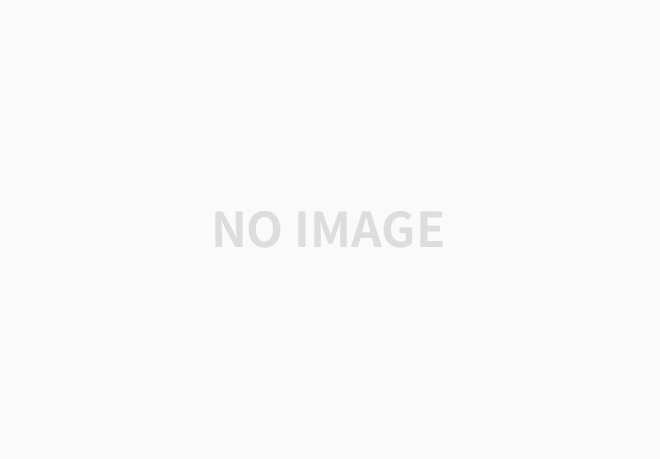
from django.shortcuts import render
from django.views.generic import TemplateView, ListView
from .models import City
# Create your views here.
class HomePageView(TemplateView):
template_name= 'home.html'
class SearchResultsView(ListView):
model = Citytemplate_name = 'search_results.html'
* Template 구조
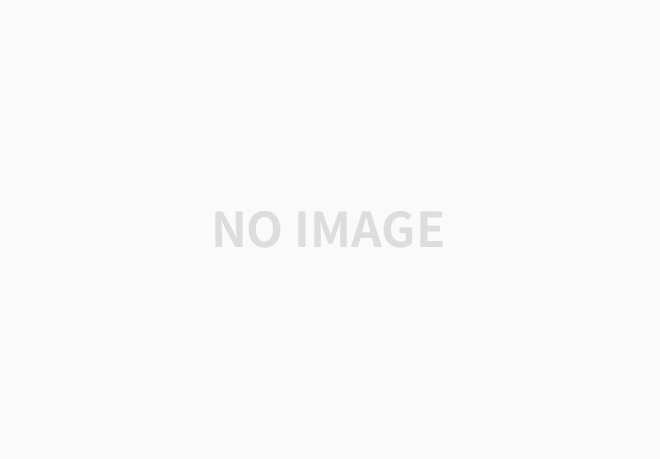
- Templates 파일을 만들어 주기
(*오타 earch_results > search_results )
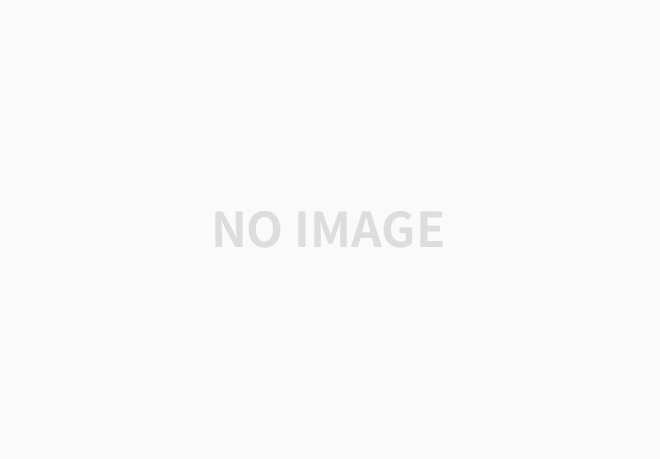
$ mkdir templates
$ touch templates/home.html
$ touch templates/search_results.html
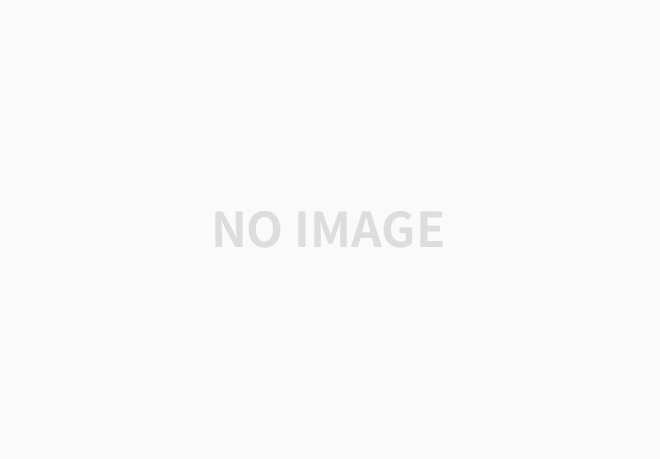
- cityserach_project/settings.py 파일에도 TEMPLATES 수정하기
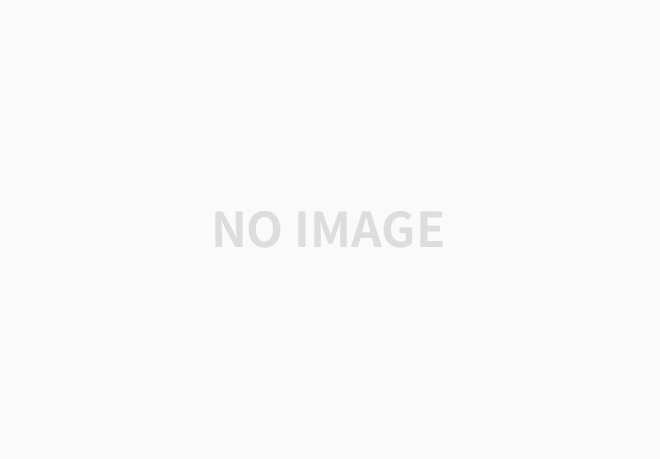
# citysearch_project/settings.py
TEMPLATES = [
{
...
'DIRS': [os.path.join(BASE_DIR, 'templates')], # new
...
}
]
- 홈페이지 창 만들기
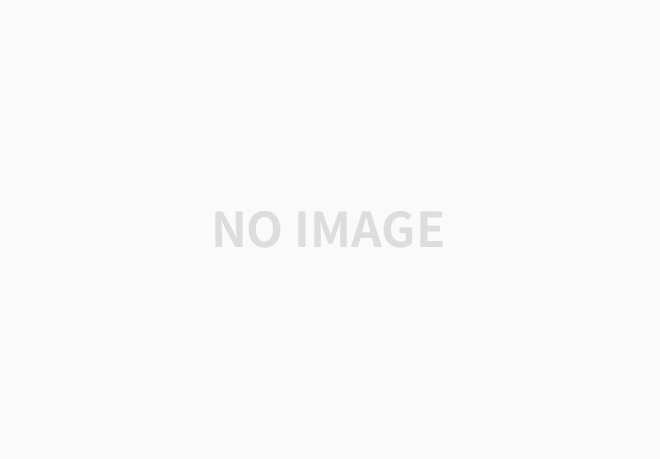
<!-- templates/home.html -->
<h1>Coogle</h1>
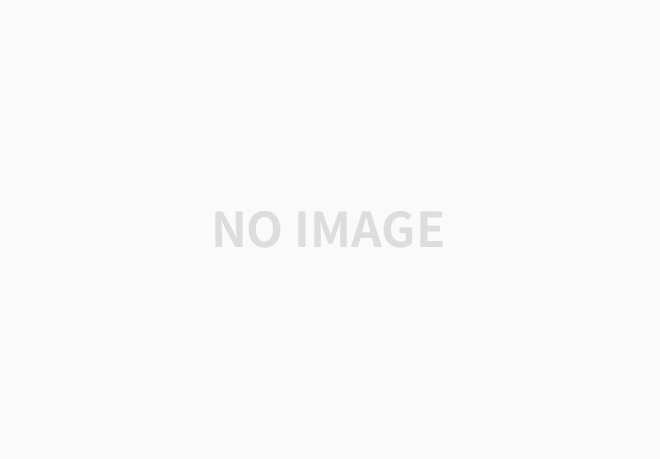
- 검색결과 창 만들기
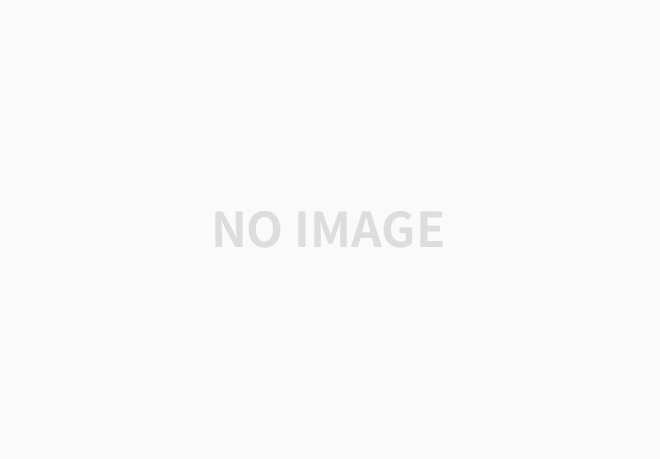
<!-- templates/search_results.html -->
<h1>Search Results</h1>
<ul>
{% for city in object_list %}
<li>
{{ city.name }}, {{ city.state }}
</li>
{% endfor %}
</ul>
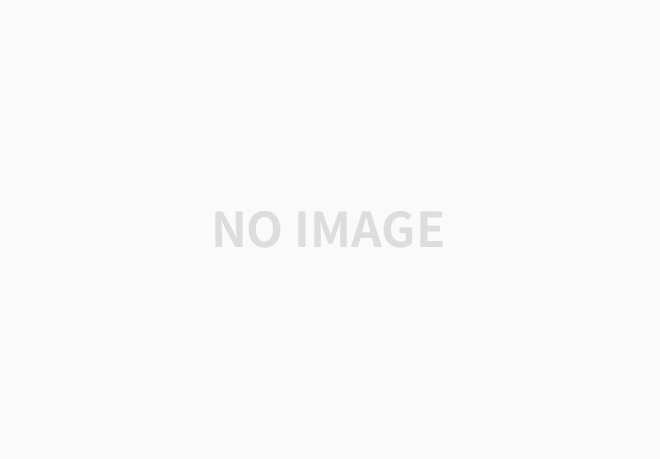
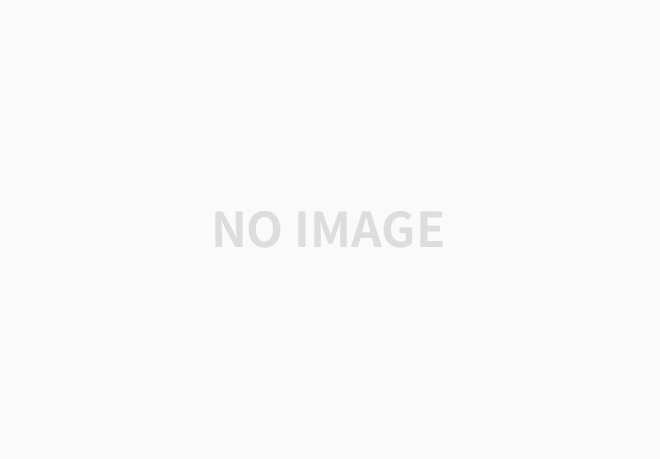
8. Basic 검색
- cities > views.py 에 get_queryset 함수 정의
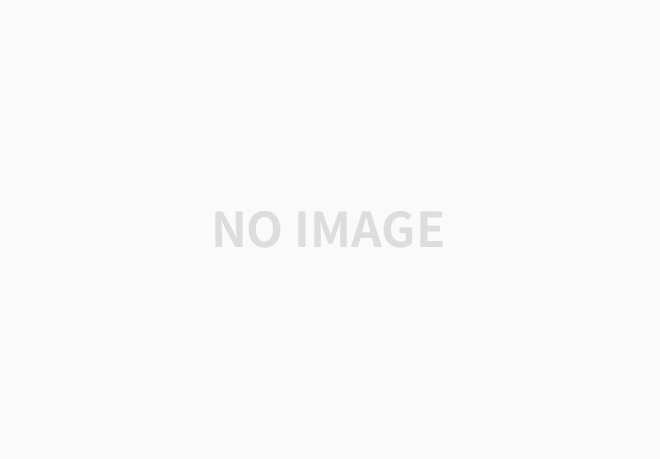
# cities/views.py
from django.shortcuts import render
from django.views.generic import TemplateView, ListView
from .models import City
# Create your views here.
class HomePageView(TemplateView):
template_name= 'home.html'
class SearchResultsView(ListView):
model = City
template_name = 'search_results.html'
def get_queryset(self): # new
return City.objects.filter(name__icontains='서울')
- name__icontains=' '에 무엇이 들어가냐에 따라 filtering 되서 표시 됌
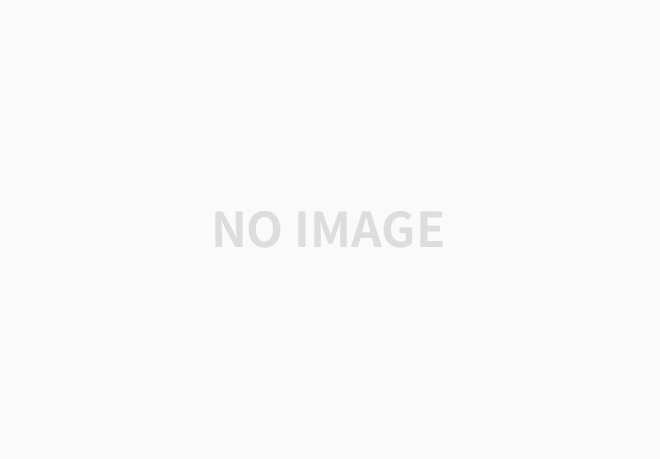
- Q option 으로 다양하게 (합집합 교집합.. 차집합.. )필터링해볼 수 있음
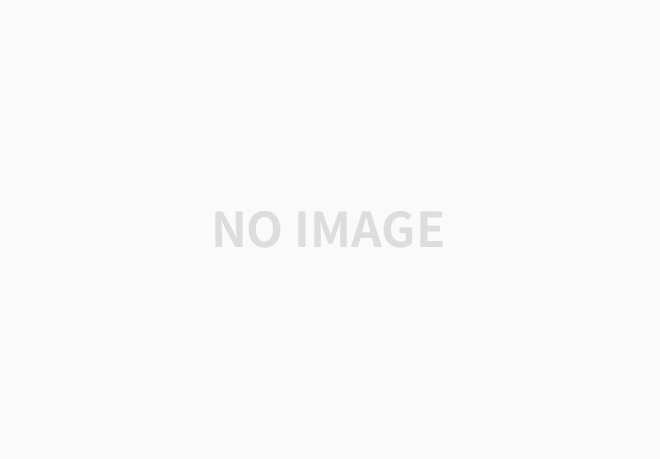
from django.shortcuts import render
from django.views.generic import TemplateView, ListView
from .models import City
from django.db.models import Q # new
# Create your views here.
class HomePageView(TemplateView):
template_name= 'home.html'
class SearchResultsView(ListView):
model = City
template_name = 'search_results.html'
def get_queryset(self): # new
return City.objects.filter(
Q(name__icontains='서울') | Q(state__icontains='한국')
)
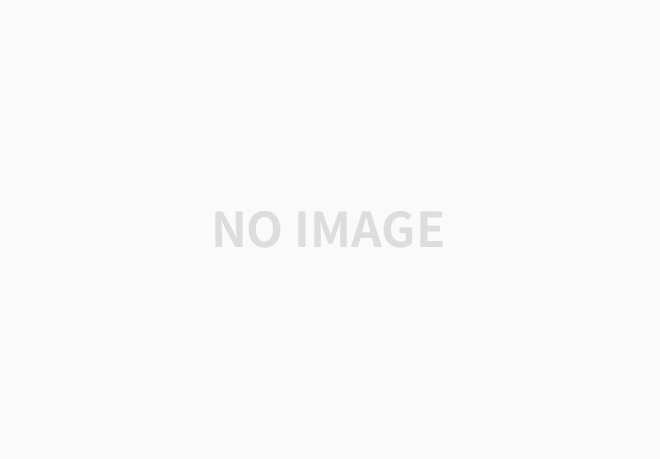
9. 검색 폼 만들기
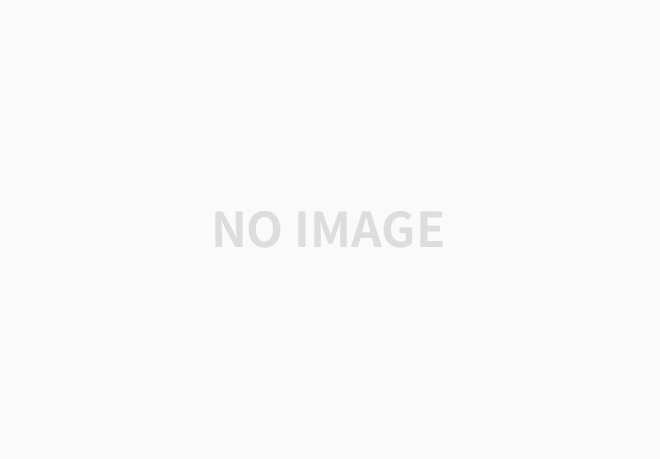
<!-- templates/home.html -->
<h1>Coogle</h1>
<!-- Search Form -->
<form action="{% url 'search_results' %}" method="get">
<input name="q" type="text" placeholder="Search...">
</form>
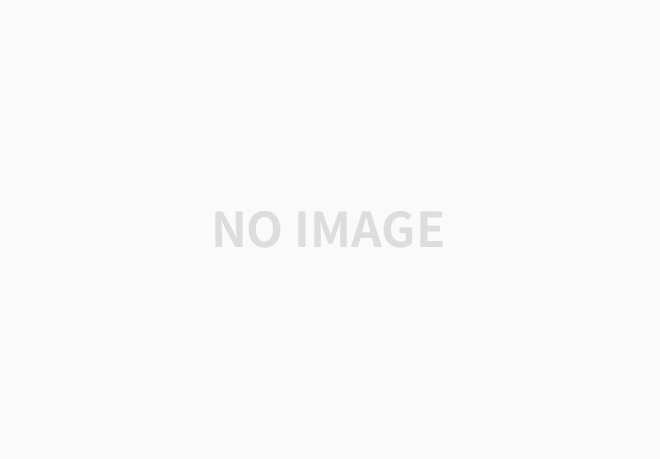
10. 검색 결과로 필터링하는 함수 만들기
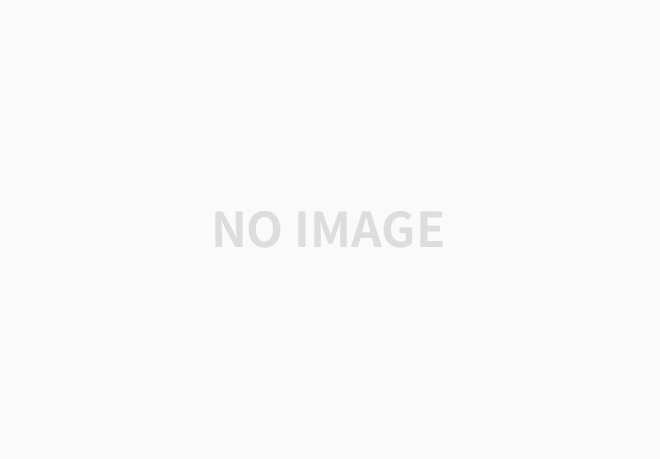
from django.shortcuts import render
from django.views.generic import TemplateView, ListView
from .models import City
from django.db.models import Q # new
# Create your views here.
class HomePageView(TemplateView):
template_name= 'home.html'
class SearchResultsView(ListView):
model = City
template_name = 'search_results.html'
def get_queryset(self): # new
query = self.request.GET.get('q')
object_list = City.objects.filter(
Q(name__icontains=query) | Q(state__icontains=query)
)
return object_list
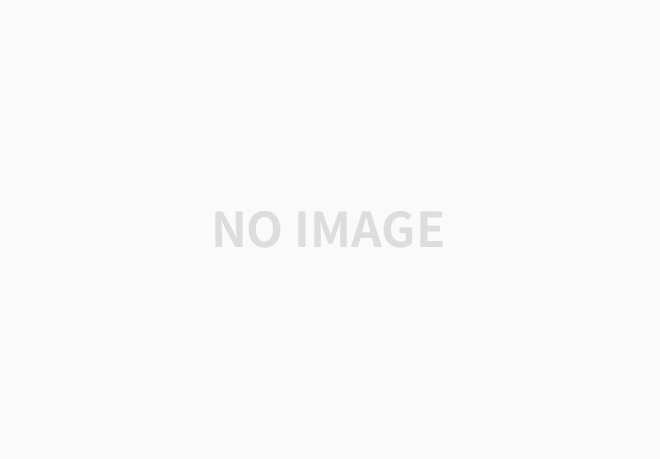
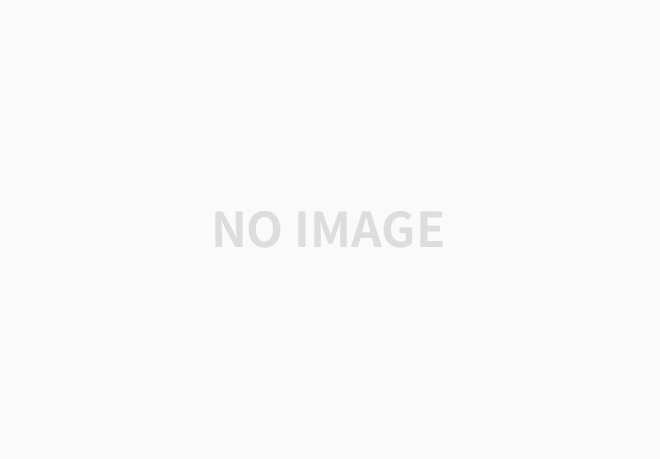
참고자료
https://learndjango.com/tutorials/django-search-tutorial
Django Search Tutorial | LearnDjango.com
Django Search Tutorial In this tutorial we will implement basic search in a Django website and touch upon ways to improve it with more advanced options. Note: I gave a version of this tutorial at DjangoCon US 2019. You can see the video here: Complete sour
learndjango.com
'노트 > Django : 웹' 카테고리의 다른 글
[웹 프로그래밍 기초] AWS를 이용하여 웹 서비스 배포하기 (0) | 2020.06.29 |
---|---|
[웹 프로그래밍 기초] 게시판에 이미지 업로드해서 불러오기 (0) | 2020.06.22 |
[웹 프로그래밍 기초] 웹페이지 댓글 기능 구현하기 (0) | 2020.06.17 |
[웹 프로그래밍 기초 ] django 관리자 계정 만들기 (0) | 2020.06.17 |
[웹 프로그래밍 기초] Django를 활용한 웹 게시판 만들기 (2) | 2020.06.15 |