[케라스] 주식가격 예측하기(2) 파이썬 코드
2020. 4. 18. 15:04ㆍ노트/Python : 프로그래밍
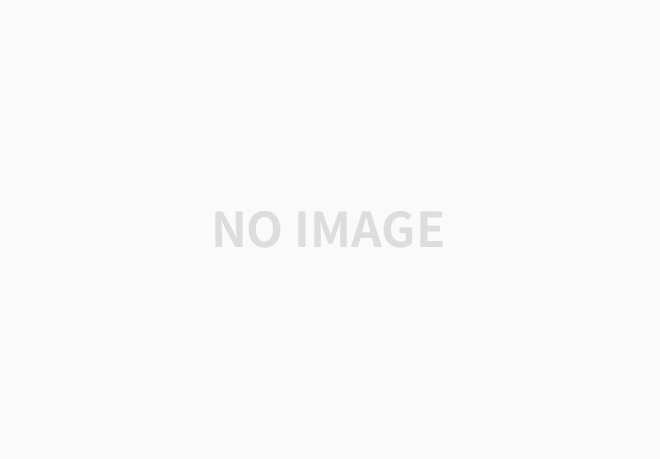
데이터
data-02-stock_daily.csv
0.04MB
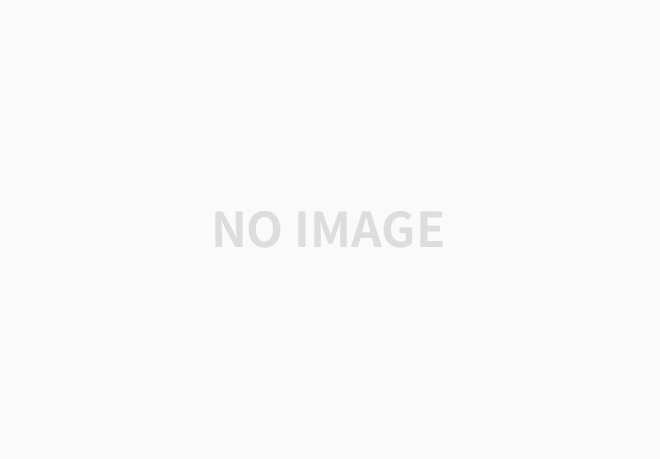
코드
# Open, High, Low , Volume으로 Close 가격 예측하기
xdata=data[["Open","High","Low","Volume"]]
ydata=pd.DataFrame(data["Close"])
# 데이터 표준화
from sklearn.preprocessing import StandardScaler
xdata_ss=StandardScaler().fit_transform(xdata)
ydata_ss=StandardScaler().fit_transform(ydata)
print(xdata_ss.shape , ydata_ss.shape)
>>>(732, 4) (732, 1)
# 트레이닝 테스트 데이터 분리
xtrain=xdata_ss[220:,:]
xtest=xdata_ss[:220,:]
ytrain=ydata_ss[220:,:]
ytest=ydata_ss[:220,:]
print( xtrain.shape , ytrain.shape , xtest.shape, ytest.shape)
>>>(512, 4) (512, 1) (220, 4) (220, 1)
모델 구성
# 모델 구성
model = Sequential()
model.add(Dense(units=1024, input_dim=4, activation='relu'))
model.add(Dense(units=512, activation='relu'))
model.add(Dense(units=256, activation='relu'))
model.add(Dense(units=128, activation='relu'))
model.add(Dense(units=64, activation='relu'))
model.add(Dense(units=32, activation='relu'))
model.add(Dense(units=1))
# 모델 학습과정 설정
model.compile(loss='mse', optimizer='adam', metrics=['mae'])
MAE는 다음과 같이 정의됩니다.
MAE=∑|y−ˆy|n
모델의 예측값과 실제값의 차이를 모두 더한다는 개념
- 절대값을 취하기 때문에 가장 직관적으로 알 수 있는 지표
- MSE 보다 특이치에 robust합니다.
- 절대값을 취하기 때문에 모델이 underperformance 인지 overperformance 인지 알 수 없습니다.
- underperformance: 모델이 실제보다 낮은 값으로 예측
- overperformance: 모델이 실제보다 높은 값으로 예측
모델 학습
# 학습 조기종료
from keras.callbacks import EarlyStopping
es = EarlyStopping(patience=10)
# 모델 트레이닝
seed = 123
np.random.seed(seed)
tf.set_random_seed(seed)
hist = model.fit(xtrain, ytrain, epochs=10, batch_size=16, callbacks=[es])
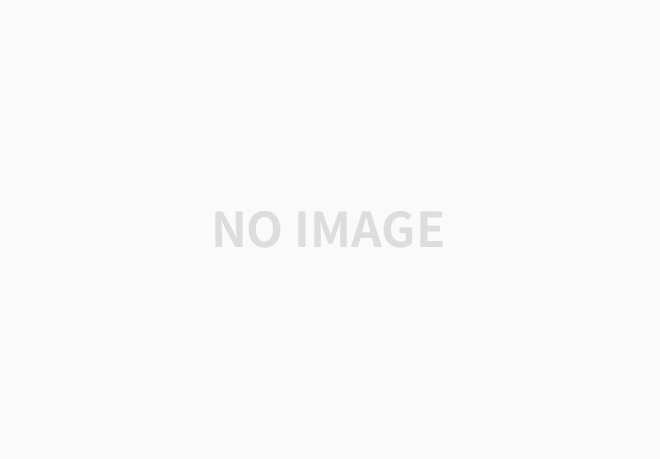
모델 검증
# 트레이닝 데이터셋으로 검증해보기 ( 원래는 검증데이터가 따로 있어야됌)
print("loss:"+ str(hist.history['loss']))
print("MAE:"+ str(hist.history['mae']))
>>>
loss:[0.10989800396782812, 0.004384054383990588, 0.004886031434580218, 0.004826842614420457, 0.003907841986801941, 0.004693856019002851, 0.005692954953701701, 0.0028650929089053534, 0.003572225868992973, 0.0034893927040684503]
MAE:[0.19797385, 0.049709965, 0.05281601, 0.048923932, 0.047958694, 0.051436342, 0.05639182, 0.040450737, 0.046064664, 0.045582384]
# 모델 테스트
res = model.evaluate(xtest, ytest, batch_size=32)
print("loss",res[0],"mae",res[1])
>>>
220/220 [==============================] - 0s 363us/step
loss 0.00187790239314464 mae 0.035955723375082016
모델 사용
#7 모델 사용
xhat = xtest
yhat = model.predict(xhat)
plt.figure()
plt.plot(yhat, label = "predicted")
plt.plot(ytest,label = "actual")
plt.legend(prop={'size': 20})
print("Evaluate : {}".format(np.average((yhat - ytest)**2)))
>>> Evaluate : 0.0018779022504846218
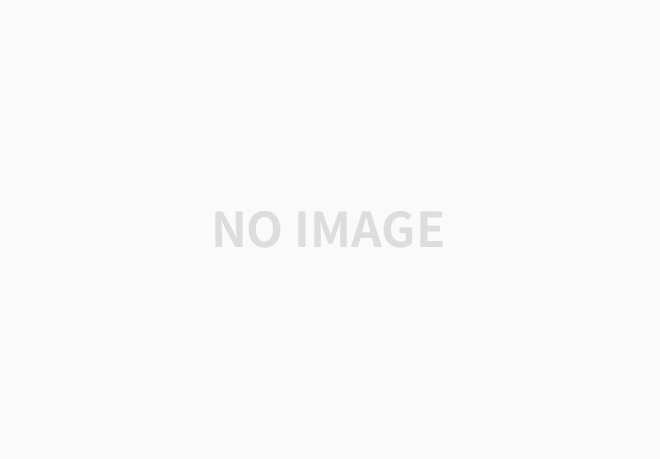
텐서플로우 버전 확인하기
2020/04/18 - [노트/Python : 프로그래밍] - [텐서플로우] 주식가격 예측하기(1) 파이썬 코드
'노트 > Python : 프로그래밍' 카테고리의 다른 글
[텐서플로우] 소프트맥스 회귀 (Softmax Regression) 분류 파이썬 코드 (0) | 2020.04.20 |
---|---|
[파이썬] 데이터시각화(1) (matplotlib.pyplot 패키지) (0) | 2020.04.20 |
[텐서플로우] 주식가격 예측하기(1) 파이썬 코드 (0) | 2020.04.18 |
[파이썬] 데이터변형 | 재구조화 (피벗테이블) (0) | 2020.04.18 |
[텐서플로우] 다중 선형 회귀를 이용한 당뇨병 분류기 파이썬 코드 (0) | 2020.04.18 |